- using function
void _changePassword(String newpassword) async{
//Create an instance of the current user.
FirebaseUser user = await FirebaseAuth.instance.currentUser();
//Pass in the password to updatePassword.
user.updatePassword(newpassword).then((_){
print("Successfully changed password");
}).catchError((error){
print("Password can't be changed" + error.toString());
//This might happen, when the wrong password is in, the user isn't found, or if the user hasn't logged in recently.
});
}
2. use of the firebase REST API way
import 'package:http/http.dart' as http;
import 'dart:convert';
import 'dart:async';
Future<Null> changePassword(String newPassword) async {
const String API_KEY = 'YOUR_API_KEY';
final String changePasswordUrl =
'https://www.googleapis.com/identitytoolkit/v3/relyingparty/setAccountInfo?key=$API_KEY';
final String idToken = await user.getIdToken(); // where user is FirebaseUser user
final Map<String, dynamic> payload = {
'email': idToken,
'password': newPassword,
'returnSecureToken': true
};
await http.post(changePasswordUrl,
body: json.encode(payload),
headers: {'Content-Type': 'application/json'},
)
}
You can get the idToken
by using the getIdToken()
method on the FirebaseUser object
You can get the firebase API key under the project setting in your console
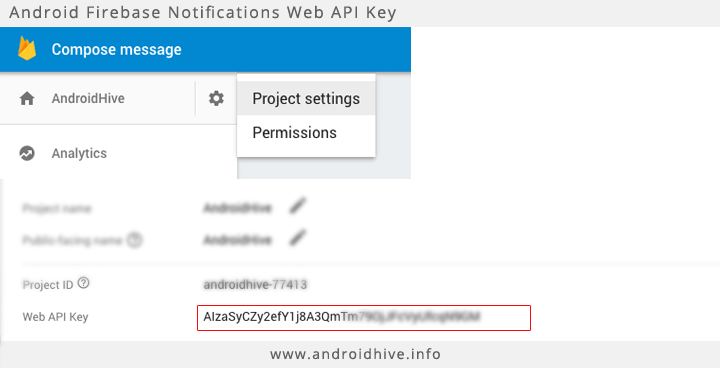